How to Create Additional Image Sizes in WordPress
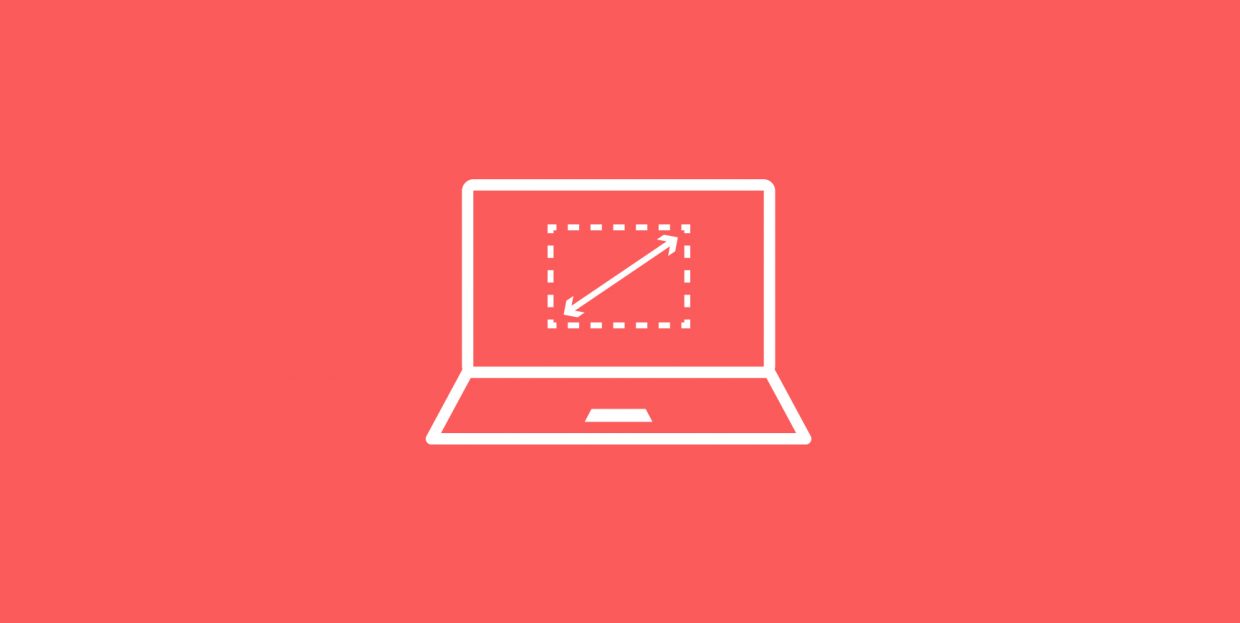
Every time you upload an image to your website’s media folder, WordPress generates various versions of that image for you to use. This lets you focus less on resizing those images yourself, and more on creating your website content. WordPress supports default, as well as custom, image size variations.
Currently, the default variations include thumbnail, medium, medium-large, large and full (the original image size). Depending on the theme and plugins you have installed, you could also use some so-called built-in image sizes. Alternatively, you can create your additional image sizes in WordPress, the process of which we’ll be explaining in this article. Please note, some parts of this article contain code, so if you find something unclear we suggest doing additional research.
With that being said, let’s proceed to dig deeper into the subject of using WordPress to add image sizes. During the course of this article, we’ll also be answering the following questions:
How to create additional image sizes in WordPress
In this section, we will cover all the required steps for creating and using an additional image size in WordPress. As some of the steps require you to add code via FTP, brushing up on your knowledge on the use of FTP might be needed. We also advise making a backup of your WordPress files, just in case.
With WordPress, we differentiate default, built-in, and additional image sizes. Defaults include thumbnail (150×150 pixels), medium (300×300 pixels), large (1024×1024 pixels), and full (original image size). There’s also a medium-large (768px in width) that was added in WordPress version 4.4. Built-in images are enabled by your theme, some of your plugins (e.g. WooCommerce), and even WordPress itself. For example, the following image sizes – 1536×1536 and 2048×2048 were added in WordPress 5.3.
Therefore, when you upload an image to your Media Library, WordPress will automatically generate several versions of that image in all the available sizes/dimensions. Provided, of course, that the original image size and orientation provide a suitable basis for the resizing. All these new versions will be kept on your server, under wp-content/uploads; they aren’t kept in your Media Library. So, when you’re adding an image to your page or post and you pick a registered image size for it, WordPress will call on the prepared version from your server.
This also means that your site can get slowed down, especially if you create multiple additional image sizes that will result in even more image versions on your server. This is why it’s particularly important to approach creating additional image sizes with three things on your mind. The first—try to upload optimally sized images in dimensions that you’ll actually be using so you avoid weighing down your server space. The second—occasionally enter your wp-content/uploads folder to delete the image versions of sizes you don’t need or use the Regenerate Thumbnails plugin to help you. And the third—limit creating custom image sizes to those you specifically plan to use.
If you want a size beyond the default options, you can register your additional image sizes using the WordPress add_image_size() function.
The add_image_size() function has four parameters: image size ID, image width in pixels, image height in pixels, and the cropping behavior. While the first three are quite straightforward, the fourth parameter this function accepts requires a bit more explanation.
If you put false as the cropping behavior (which is the default value of the parameter), you will enable soft image cropping. Soft cropping scales the image down until it fits the specified dimensions (or at least one of the dimensions) while maintaining the original aspect ratio. As such, the term resizing downwards is probably the more appropriate even though soft cropping is still used.
On the other hand, if you specifically put true as the cropping behavior, then you will enable hard image cropping. This will crop the image to the specified dimensions using the center of the original image for positioning. You can specify the cropping position by inserting an array with the x and y-axis cropping position. The accepted x-axis values are ‘left’, ‘center’ or ‘right’, while the accepted y-axis values are ‘top’, ‘center’ or ‘bottom’.
Here’s an example of how you can use the add_image_size function for hard cropping:
add_image_size( 'your-custom-size', 700, 500, array( 'left', 'top' ) );
In this example, the image size identifier is called your-custom-size, the image size is 700×500 and the image is hard-cropped in the top-left position. Simply put, your new image will take up 700×500 pixels of the original image’s top left section.
But, before we show the example we made for this article, we need to add two important notices.
-
Both types of cropping are only possible if the uploaded image is greater in size than the specified image dimensions. Meaning, if the original image is too small, it is not possible to crop or rescale it into a smaller image. This is true for any image size except full, as it retains the original image size. As such, make sure to upload the image in dimensions greater than those you wish to create from that image.
-
A prerequisite to adding additional image sizes is to enable support for post thumbnails on the theme you are using. This is done by adding the following bit of code in the functions.php file of your theme before calling the add_image_size() function:
add_theme_support( 'post-thumbnails' );
Knowing that, we can proceed to our actual example. The proper places for adding any code are the functions.php file of your theme or inside a site-specific plugin. When creating this article, we have opted for the former. However, we won’t be going into detail on how to insert this code to either of the suggested places as the location is a matter of individual preference. Instead, we will focus on explaining the code in detail. Needless to say, you can change any of the arguments of the add_image_size() function, as the ones we used were only examples.
Our code represents a custom-made function called register_custom_image_sizes that is hooked onto the after_setup_theme hook using the add_action() function. The register_custom_image_sizes function contains two parts. One conditional part is for adding theme support for thumbnails and the other part is for registering the two new image sizes. The conditional part adds the support for post thumbnails only if it wasn’t previously added to your theme. The two image sizes we will register have custom-small-square and custom-landscape as their IDs. The dimensions of the former are 450×450 and it is hard-cropped in the central position, while the latter has the dimensions 1000×600 and it is soft-cropped.
function register_custom_image_sizes() { if ( ! current_theme_supports( 'post-thumbnails' ) ) { add_theme_support( 'post-thumbnails' ); } add_image_size( 'custom-small-square', 450, 450, true ); add_image_size( 'custom-landscape', 1000, 600 ); } add_action( 'after_setup_theme', 'register_custom_image_sizes' );
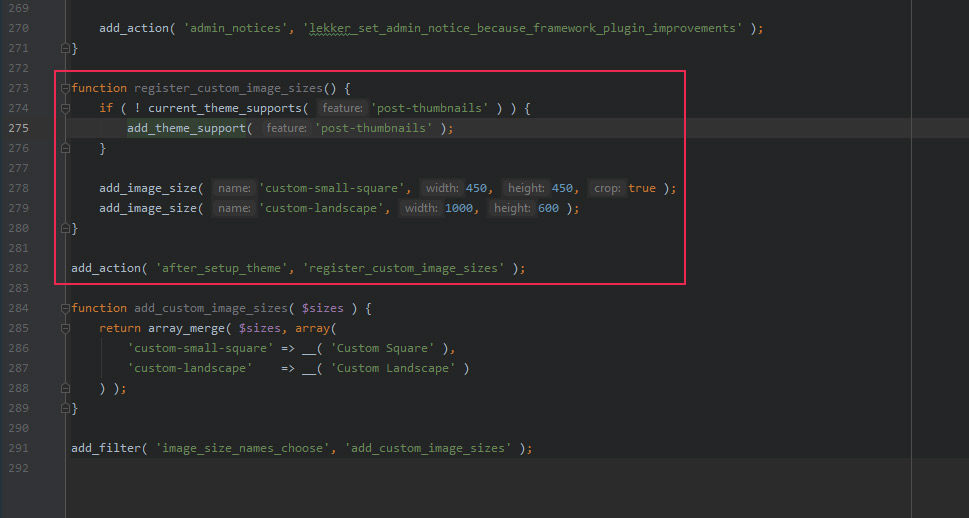
Following this example code, you can register any image sizes you like. But, make sure to replace the arguments of the add_image_size() functions with ones that best suit your website.
After registering the new image sizes, you are ready to use them inside your theme. To do so, you would need to edit the corresponding template file with a piece of code that calls one of the added custom image sizes. While that approach is more suited for advanced WordPress users, there is a way to enable a more user-friendly approach. It involves the option to choose the added custom image size for any image added with a WordPress page builder on your page or post. Even though there are WordPress plugins specifically made for this purpose, we have opted to show how to enable this functionality with a simple code snippet as it’s more reliable. As we mentioned earlier, you can either add this snippet to the functions.php file of your theme or inside a site-specific plugin.
The code is very simple – the add_custom_image_sizes() function that we created is hooked onto an image_size_names_choose filter. The function adds the two newly created image sizes to the array of existing image sizes. The names of those two image sizes are set to Custom Square and Custom Landscape, respectively.
function add_custom_image_sizes( $sizes ) { return array_merge( $sizes, array( 'custom-small-square' => __( 'Custom Square' ), 'custom-landscape' => __( 'Custom Landscape' ) ) ); } add_filter( 'image_size_names_choose', 'add_custom_image_sizes' );
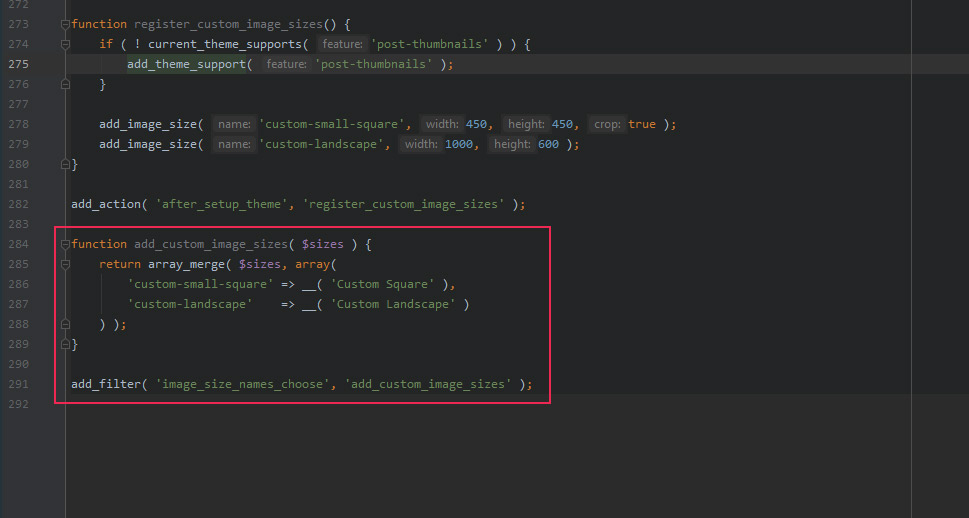
After adding this code, in the next part of the text, we will explore how to use the added image sizes.
One way of using the additional image sizes is to add code inside the appropriate theme file. The question of which file you need to use depends on two things—the theme’s file structure and where exactly (in which part of the website) you wish to display the image. There are also a couple of different functions you can consider using for adding your additional image size. One of them is the_post_thumbnail() function. It accepts up to two parameters—image size and additional attributes. The image size could be represented using a valid image size identifier or as an array of dimensions, meaning width and height, in pixels. The additional attributes could be a query string or an array of attributes that you wish to add to the image. When displaying images of custom image sizes using this function you should have something that looks like this:
the_post_thumbnail( 'your-custom-size' );
Please note, the part with your-custom-size should be replaced with a registered image size identifier. In our example, those would be custom-small-square or custom-landscape. Also, this function should be used inside a WordPress Loop.
Another function that is very similar to the one above and that you can consider using is the get_the_post_thumbnail() function. Besides image size and additional attributes, it accepts one more parameter—post ID or WP_Post object. You’d add it the same way you would the_post_thumbnail() function.
But, as not all WordPress users are comfortable with code, we will show one more way of using additional image sizes on your site. This is managed by choosing them for the appropriate image elements within your chosen editor/page builder plugin. In the following section, we will show you how to do this for Elementor, WPBakery, and Gutenberg.
If you are using Elementor as your page builder, you can add an Image widget and choose its image size from the appropriately named option within the Content tab. The list will contain all the image sizes, including the two we added. Additionally, Elementor has a custom option that allows you to specify the exact dimensions in which you want the image to appear.
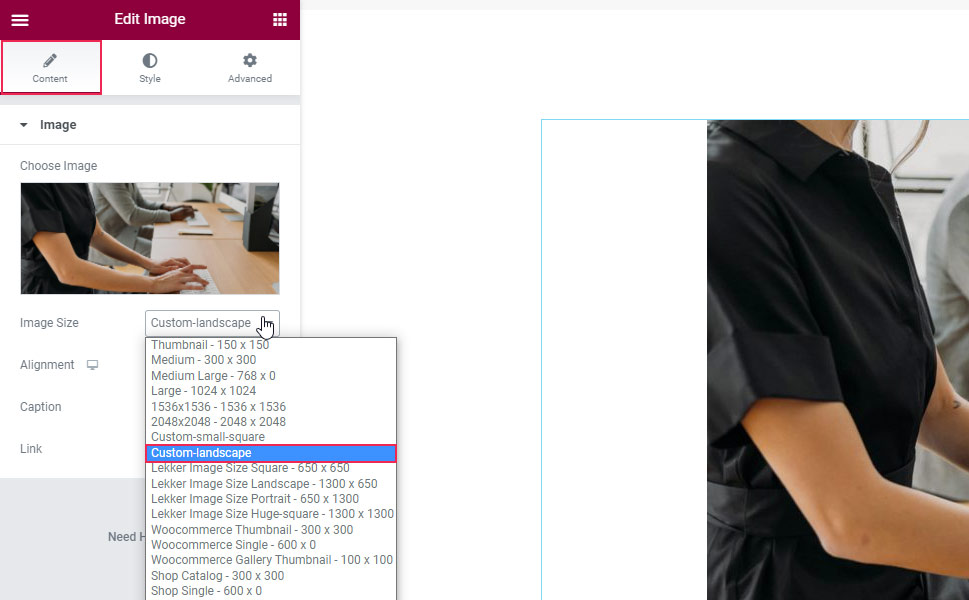
The procedure is very similar if you are using WPBakery as your page builder. You can add a Single Image shortcode, which has an option for inputting image sizes. That option accepts image size IDs, which can be default, built-in, or ones you added yourself. At the same time, it retains the possibility to specify the exact dimensions of the image in pixels.
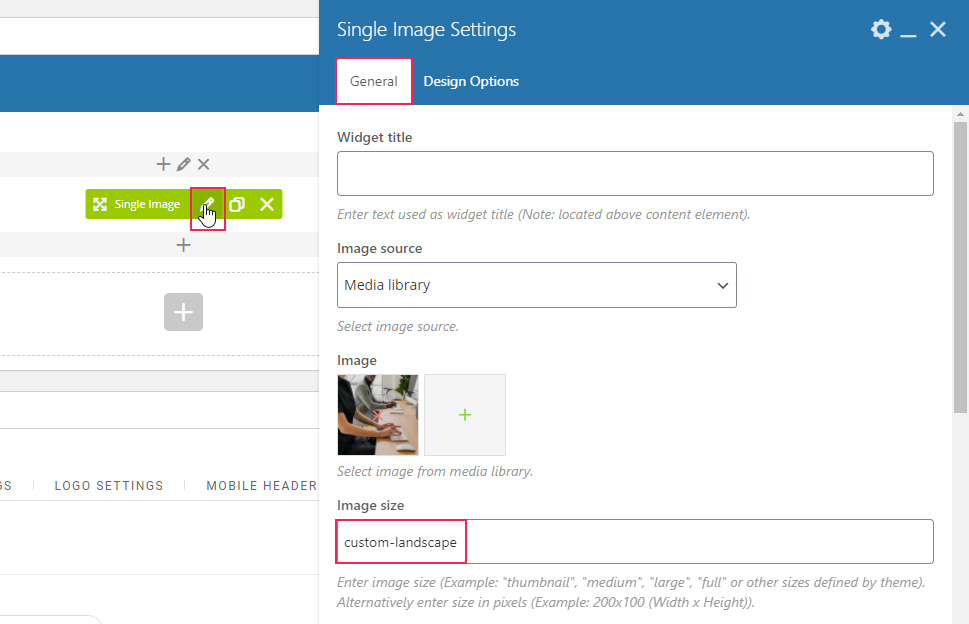
On the other hand, if you are using Gutenberg as your page builder, the appropriate element is called an image block. Then, while having it selected, you can choose an image size from the dropdown on the right side of the screen. The available image sizes are the default ones (though they don’t include medium-large) and the custom sizes that we added. Gutenberg also has separate options for specifying the exact dimensions of the image, including the four resizing options: 25%, 50%, 75%, and 100%.
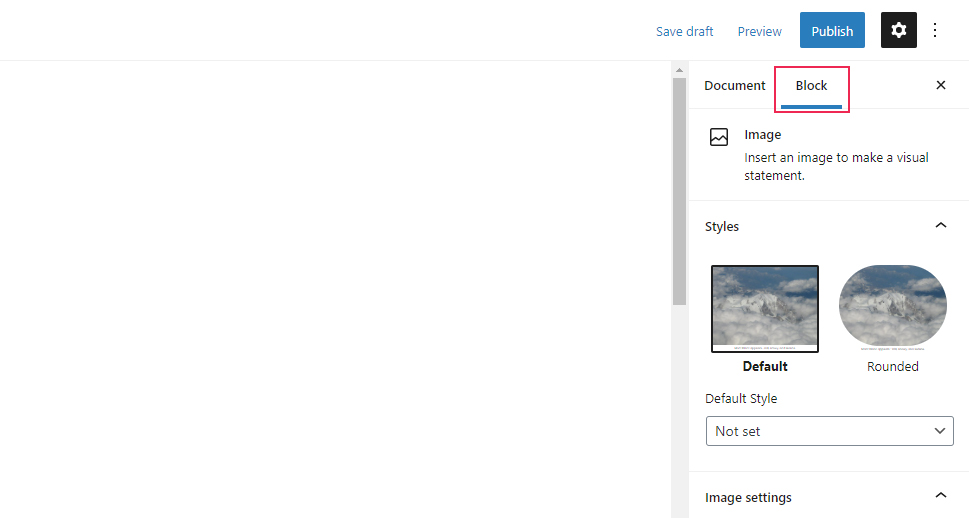
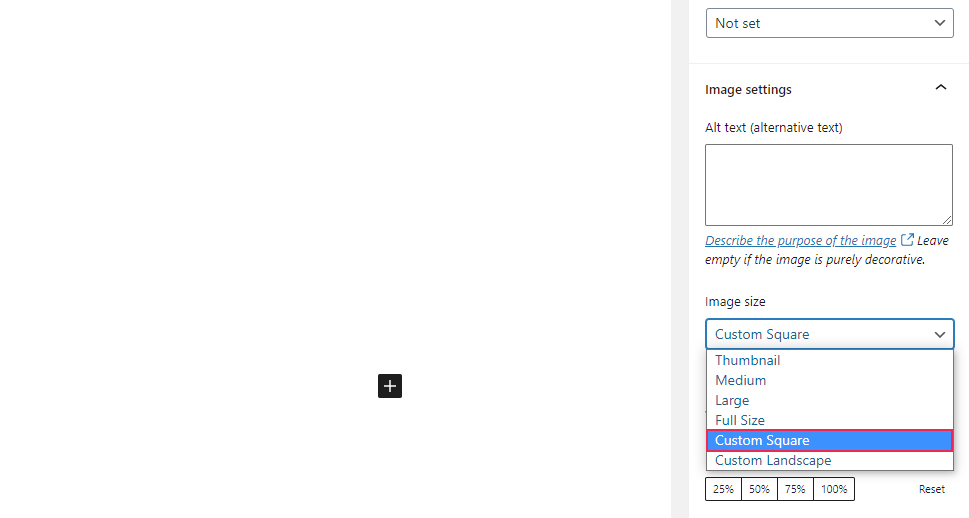
This concludes the main part of our guide on how you can create and use additional image sizes in WordPress. In the following section, we will cover a very important last step that ensures the custom image size functionality—image size regeneration.
It is important to know that the code for adding custom image sizes works only on images that are uploaded after the code is added. This is because when you upload an image, all registered image size versions of that image are created. That’s possible as long as the original image has sufficient dimensions. However, the process doesn’t work retroactively. Meaning, after you create an additional image size, the existing images won’t automatically get a new version in the added size. Neither the code nor the page builder options will directly change the image size of an existing image in your content to a newly created size. Therefore, for the whole process to work on your old images, you would need to create new image versions for them, i.e. to regenerate your existing images.
If you ever decide to remove an existing image size, you should remove all the image versions corresponding to that image size to save server space. It’s important to do this when switching themes, or when changing the dimensions of the existing image size inside your admin panel using Settings > Media.
Luckily, there are a few WordPress plugins that fulfill all the requirements given above. The most popular of them is the Regenerate Thumbnails plugin. It is a very straightforward plugin made specifically for image regeneration.
After you install the plugin, navigate to the Tools > Regenerate Thumbnails section. On that page, you will see a list of your currently available thumbnail sizes, as well as two buttons. Press the Regenerate Thumbnails For All X Attachments button, where the X will be replaced with the number of attachments you have.
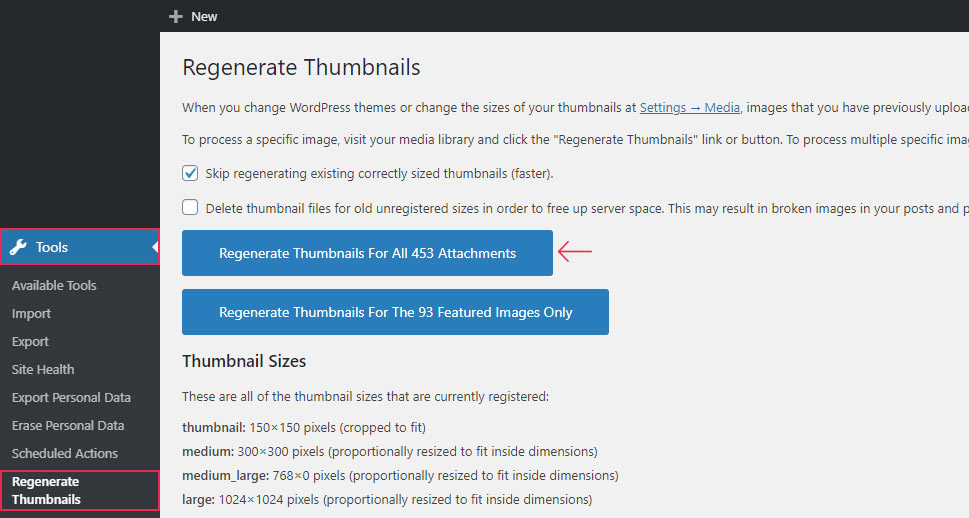
Depending on the number of attachments, the process of regeneration could take quite a while. It is worth noting that there are two additional checkboxes you can select before pressing the Regenerate Thumbnails button. Those options are for not regenerating images that are already correctly sized and for deleting image versions of unregistered image sizes. For now, we have opted to leave them set to their default values.
Additionally, if you don’t want to regenerate all your images in bulk, you can access a single image in your Media library and regenerate it by pressing the Regenerate Thumbnails button found in the bottom right corner. To regenerate only specific images you want, repeat this process for them one by one.
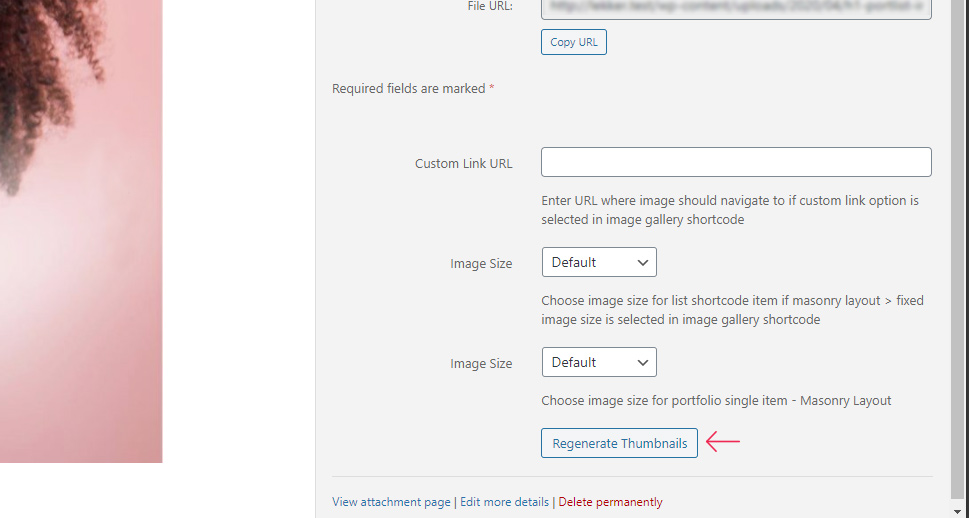
However, if you opted for bulk regeneration, you will be able to see the progress bar and a list of regenerated images in the top section of the screen. Wait for the process to complete, after which you will get a success message that will look like the one shown below.
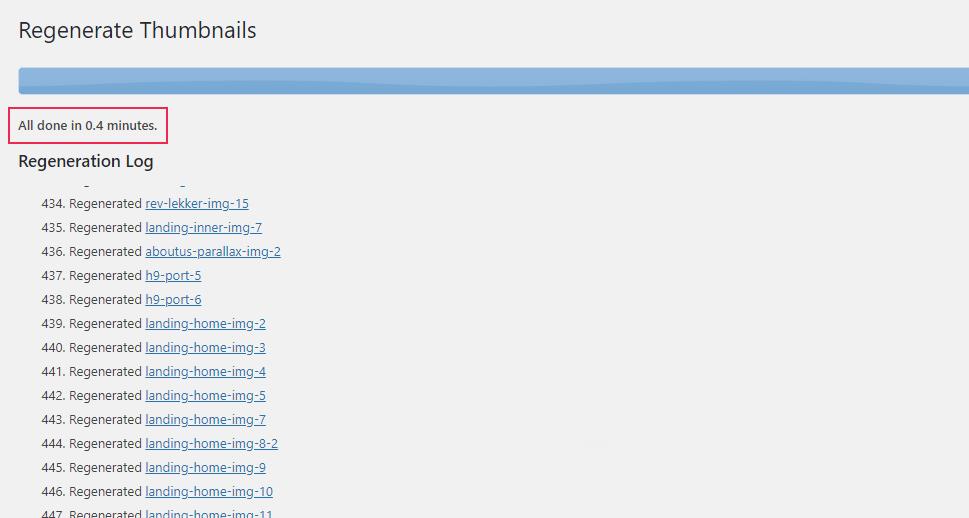
Final Thoughts
Creating custom image sizes in WordPress ensures the optimal display of images across your website while having a positive impact on your website speed. As such, knowing how to use WordPress to add an image size is an important asset for any webmaster. Luckily, the process can be broken down into easy-to-understand steps, which we have covered in detail in this article. And some of the advice we provided could optimize the use of images across your website. Therefore, we highly advise bookmarking this article, as it could keep proving useful for a variety of reasons.