How to Create a Custom Typeout Effect Using Code
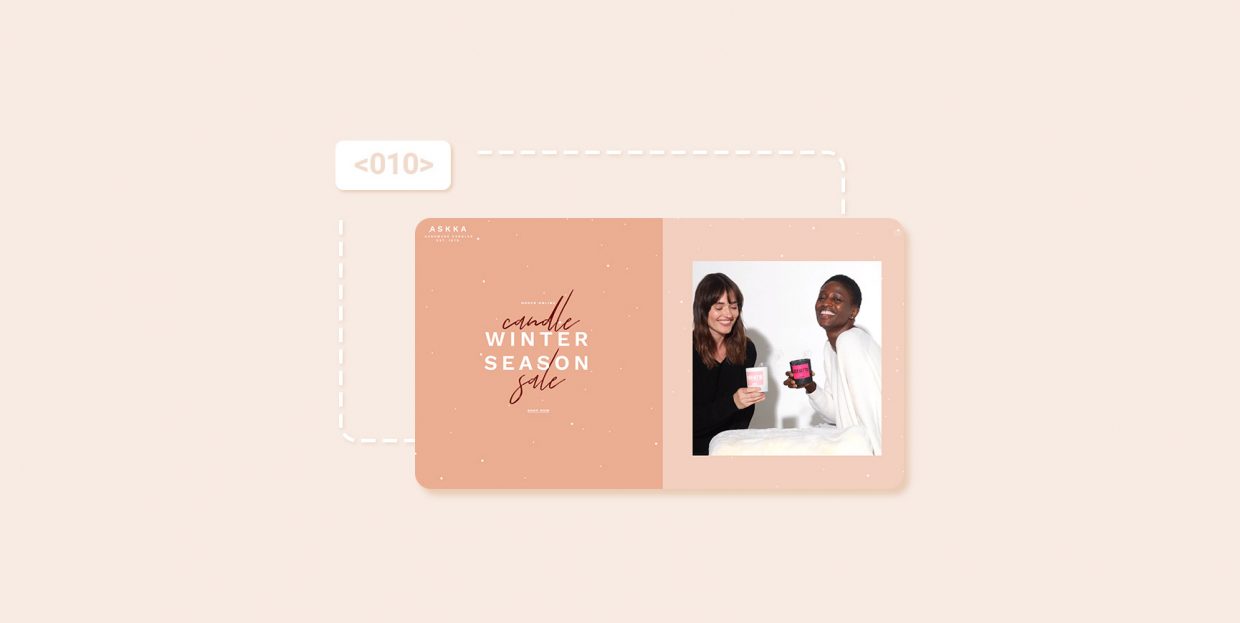
Making your site stand out from the competition can be difficult. That’s why an engaging feature that will attract visitors and entice them to stay is key. And very little can beat animated effects when it comes to visitor engagement. One example of this is the animated typeout text effect. With it, you can create sections of text that are seemingly being typed out in real-time. The typeout text effect is neat enough to stand on its own, but you can also combine it with some static text to achieve different looks.
In this article, we’ll take a look at how an animated typeout text effect is made and how to add it to your WordPress website.
How to create a custom typeout effect using code
There are two ways you can add a custom typeout effect in WordPress—using a suitable WordPress plugin or with custom code. Using a plugin is the more beginner-friendly option, so if that’s what you decide to do, we invite you to try the Qi Addons for Elementor plugin, which was created by the same team behind our award-winning WordPress themes. Qi Addons is a free library of 60 flexible Elementor widgets, i.e. add-ons, that let you customize your WordPress website with ease. It contains Elementor addons that span a range of areas from business, infographic, presentational, creative, WooCommerce to typography, SEO, and form style. Within the typography category, you’ll find the Typeout Text widget, which offers a simple way of adding typeout effects to your website.
However, our focus in this article will be how to add a custom typeout effect with code. This method is more suitable for intermediate and advanced WordPress users, as it requires familiarity with CSS and/or JS code. The benefit of using it is that you can customize all aspects of the typeout effect exactly according to your needs. And, in case you’re just curious to see how the typeout effect works “under the hood”, we’ll be showing you the process using code created specifically for this article.
With that being said, before we move on to the how-to part of this article, we suggest you make a backup of your website beforehand. This is just a precautionary measure to ensure its safety. Once you’ve done that, you can proceed to the first part of the code, which is shown below.
function custom_typing_text( $atts ) { $default_atts = array( "text" => '', "text_color" => 'black' ); $params = shortcode_atts( $default_atts, $atts ); $text_styles = ""; if( ! empty( $params['text_color'] ) ) { $text_styles .= "color: " . $params['text_color'] . ";"; } return '<div class="text-holder"><span style="' . $text_styles . '">' . esc_html( $params['text'] ) . '</span></div>'; } add_shortcode( 'custom-typing-text', 'custom_typing_text' );
This code represents a very simple custom shortcode that we named custom-typing-text. It was registered using the add_shortcode() function with a callback function named custom_typing_text() that we created for it. The shortcode accepts two parameters—text and text_color. Its output is the text you add, colored in the text color you set.
More specifically, the text is wrapped in a <span> tag, with the color added using inline CSS. Additionally, if a user forgets to set the text_color property, it will default to the black color. The <span> is also wrapped with a <div> element, which has a custom class called text-holder. The custom class is added to help with creating CSS code that will be specific to it.
To summarize, the shortcode above is created to display a text in a given color only. To use this code, you will need to add it to the functions.php file of your child theme, using FTP or within a site-specific plugin.
To create a custom typeout effect, besides using the previously created shortcode, you will need to add additional code to animate the text that is displayed. Generally speaking, the typeout effect can be created using either JS and/or CSS code. In this article, we’ll explain how to do it using CSS only. The example we prepared is relatively simple but it should be enough to illustrate how the effect is achieved. You can use this CSS as is or you can modify it to fit your needs. With that being said, you can find the CSS code that we created below.
.text-holder { display: -webkit-inline-box; display: -ms-inline-flexbox; display: inline-flex; -webkit-box-pack: center; -ms-flex-pack: center; justify-content: center; } .text-holder span { font-size: 25px; letter-spacing: 0.2em; border-right-width: 3px; border-right-style: solid; overflow: hidden; white-space: nowrap; -webkit-animation: typing-animation 7s steps(30, end) infinite, blink-animation .5s step-end infinite; animation: typing-animation 7s steps(30, end) infinite, blink-animation .5s step-end infinite; } /* The typing effect */ @-webkit-keyframes typing-animation { from, to { width: 0 } 50% { width: 100% } } @keyframes typing-animation { from, to { width: 0 } 50% { width: 100% } } /* The blinking cursor effect */ @-webkit-keyframes blink-animation { from, to { border-color: transparent } 50% { border-color: #000 } } @keyframes blink-animation { from, to { border-color: transparent } 50% { border-color: #000 } }
Let’s break this code down.
First of all, we applied two CSS rules to the <div> element that wraps the text. By setting the display to inline-flex, we made the element inline and its inner <span> element, which contains the text, flexible. Also, the <span> is justified starting from the center of the <div> element container using the justify-content: center; rule. Using this CSS property will help with the animations’ appearance—it will create a delicate sliding effect as the text is being revealed, or typed out. All of this covered by the portion of the code you can see below:
.text-holder { display: -webkit-inline-box; display: -ms-inline-flexbox; display: inline-flex; -webkit-box-pack: center; -ms-flex-pack: center; justify-content: center; }
The second part of the CSS contains the rules applied directly to the <span> element that holds the inserted text. We set the font size and line spacing for the text, and added a 3px thick border to the right of the text. This border will imitate a blinking cursor that would go with the typing animation. The border color is specified in the section that contains the two custom animations, which we created and applied to the <span> element.
Apart from the animation code, there are two other lines of code that we need to touch on. The overflow: hidden; rule makes sure the text is hidden before the animations start. And the white-space: nowrap; rule keeps the <span> text within a single line.
All of this covered by the portion of the code you can see below:
.text-holder span { font-size: 25px; letter-spacing: 0.2em; border-right-width: 3px; border-right-style: solid; overflow: hidden; white-space: nowrap; }
Now we come to the part with the animation code. For this article, we created two simple custom animations called the typing-animation and the blink-animation using the @keyframes CSS rule. In its first half, the typing-animation gradually increases the width of the span containing the text from 0% up to 100%. In the second half of the animation, the width that was reached is gradually shrunk back down to 0%. This lets the animation mimic the writing of text and its subsequent erasure. The blink-animation mimics the blinking of the right border that we are using as the cursor. The blinking effect is created by gradually changing the border color from transparent to black and back again.
This covered by the portion of the code you can see below:
/* The typing effect */ @-webkit-keyframes typing-animation { from, to { width: 0 } 50% { width: 100% } } @keyframes typing-animation { from, to { width: 0 } 50% { width: 100% } } /* The blinking cursor effect */ @-webkit-keyframes blink-animation { from, to { border-color: transparent } 50% { border-color: #000 } } @keyframes blink-animation { from, to { border-color: transparent } 50% { border-color: #000 } }
We applied both of these animations to our <span> element using the animation shorthand CSS property. The animation shorthand has several properties but we only used a few. First, we called the typing-animation property with the duration of 7 seconds and set the animation-timing-function property to steps(30, end), which means the typing effect is broken into 30 steps, with the last step matching the end of the animation.
Setting the animation-iteration-count property to infinite results in the text constantly being “written” and “erased”. As for the blink-animation, its duration is 0.5 seconds, the animation-timing function is set to step-end and the animation-iteration-count property is also set to infinite, so it repeats endlessly. Since step-end is equal to steps(1, end), it means that the change in the color of the right border is far less gradual than it otherwise would be. This creates the impression of the “cursor” blinking rather than fading in and out.
All of this covered by the portion of the code you can see below:
.text-holder span { -webkit-animation: typing-animation 7s steps(30, end) infinite, blink-animation .5s step-end infinite; animation: typing-animation 7s steps(30, end) infinite, blink-animation .5s step-end infinite; }
This concludes our explanation of the CSS code we created. Now the question remains—where do you add it?
When working with CSS code like the one above or any similar to it, you can always add it under Appearance > Customize > Additional CSS. This is the default location for inserting website-related CSS and WordPress users should place their CSS code there. However, more advanced WordPress users can opt to put their CSS in a separate file and enqueue it using the wp_enqueue_style() function. If you’d like to learn more on this topic, you can check out our article on enqueueing scripts and stylesheets.
Now, let’s examine how this custom typeout text shortcode is used.
Custom shortcodes can be inserted into pages and posts by using the appropriate shortcode call with the necessary parameters that the shortcode supports. Since our custom shortcode has the name custom-typing-text and supports two parameters – text and text_color, an example of a proper shortcode call would be the following:
[custom-typing-text text="your-text-here" text_color="your-text-color-here"]
Don’t forget to replace the dummy properties for both the text and text color before using the shortcode call. Once you’ve done that, for the shortcode to display on a page, you need to insert the call into an appropriate shortcode-rendering element of your page builder plugin. For example, that could be the Text Block shortcode, if you are using the WPBakery plugin. But, if you are using Elementor, then you’ll need the Text Editor widget. And for Gutenberg users, the Shortcode block is the appropriate element.
If you need any further help with how to use and add your custom shortcode, you can check out our article on creating custom shortcodes. You’ll find extensive coverage of anything you might need there. For the sake of brevity, we will only show the use of a custom shortcode with the Gutenberg editor below.
To use this shortcode with the Gutenberg editor, click on the “+” icon to add a new block and then find the Shortcode block. Next, click on the Shortcode block to add it and insert your (previously customized) shortcode call inside it. After that, you can update the page and examine the results. At this point, you can also include additional content on the page. As you can see from the screenshot below, we have added a small paragraph above the shortcode call.
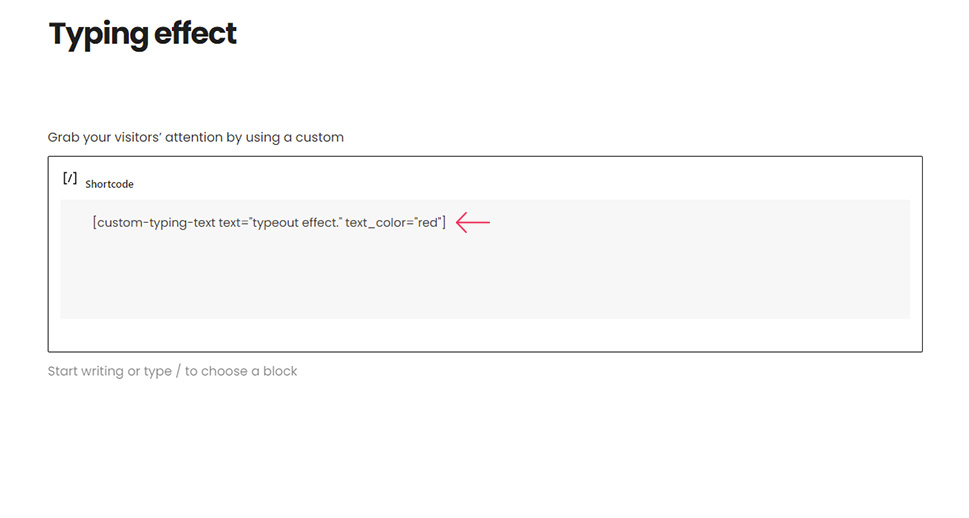
This got us the following result.
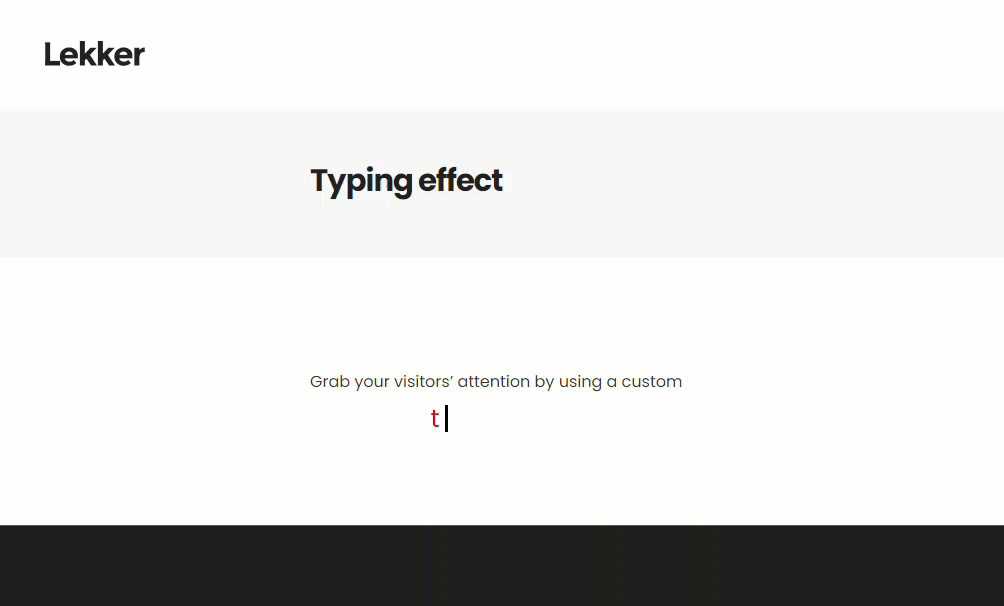
Finally, you should keep in mind that the example we created is just one of many ways how a typeout effect can be implemented using code. We showcased the method that uses CSS and shared the code that we used to create the effect. However, this example CSS might not fit the design requirements of a different WordPress website. For example, the fonts and the color scheme on your site could clash with the ones we used in the code.
In that case, you will need to customize the CSS code to match your website design or even alter the animation code if needed. Since we took care to explain every line of code carefully, we are confident that you will be able to implement the necessary modifications easily. And, to ensure your code remains cross-browser compatible, take care to change the part of the CSS code that contains the CSS vendor prefixes.
And, if you aren’t very familiar with CSS, the typeout effect is a neat way to learn something new, gain confidence, and enhance your site at the same time. There is a wealth of online resources available to help you, and you can always take a peek at our tutorials section.
Final Thoughts
The typeout text is one of the easiest ways of appealing to visitors and increasing their engagement with your WordPress website. This makes it a neat trick every WordPress owner should know. In this article, we discussed how you can implement the typeout effect using code. To illustrate how this is done, we created a custom shortcode as well as the CSS code that enables the typeout effect. Now all you need to do is follow along with the explanations and figure out which bits of the provided code you’d need to customize to make it fit with your site design.
However, if you find yourself stuck or unsure how to edit the code appropriately, you can always use a plugin to add the typeout effect to your site. This is, actually, the preferred choice of many WordPress users who are new to site design or aren’t confident about working with code. If you choose to use a plugin, we recommend the Qi Addons for Elementor and its Typeout Text widget. The typeout text effect can be a powerful tool for helping your website stand out, so make sure not to miss out on it either way.